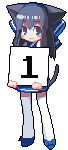
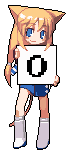
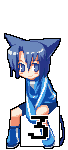
status bar (binstatus) changes
12/12/2019
I've rewritten my status bar that I use for dwm. It is now completely dynamic and allows customisation through a config.h header file. Yes, I am copying the suckless way of doing things - it's great.
The bar looks like this currently:
(time, battery percent, battery status - D for discharging battery)
The status bar is customised by changing an array that consists of structs. The struct used contains a pointer to a function and a flag (integer). You can use any of the provided functions (see components.h) as a part of the struct. Adding your own is easy, they must just return a char *.
static const struct component components[] ={ /* function flag */ {currenttime, NORMALTIME|SHOWMERIDIEM}, {battery, NONE}, {charging, NONE}, };The image above was made using this array. If you so desire, you can add more clocks, or any other component, just add another array element. The components are drawn in order.
Flags currently include: 12 hour time, 24 hour time, time shown in binary and whether to show AM/PM. (OR the flags together)
The source code can be found in my git repo.
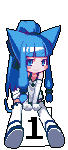
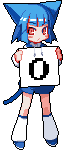
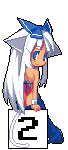
Got some things
3/12/2019
I've purchased a few cool things lately:
I finally got a thinkpad x200! A friend found the listing on ebay - it was a reasonable price compared to everything else available. 4GB ram, no damage, some scratches on the lid. I am yet to libreboot it, but that is the plan. There will be a post on doing that at some point.
I have purchased a new SSD for my desktop, so this x200 will eventually get the SSD that was in that. Or I will just buy a cheap one. Honestly, the HDD is fast enough for me.
I also got some figures, wall scrolls and a boxset. The pictures are shit and don't represent real colors and detail... The yagate scroll is shit in real life, though.
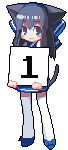
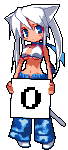
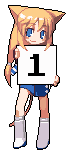
Mute music while something else plays audio
1/12/2019
I wanted to mute my music when another application (mpv or browser) started playing an audio stream and unmute the music when that stream ended. To do this I had a look at the pulseaudio API and started investigating how I could go about doing this. Quickly someone pointed me to the modules page on the pulseaudio wiki, specifically the role ducking module.
"This module lowers the volume of less important streams when a more important stream appears, and raises the volume back up once the important stream has finished (this is called "ducking")."
This is exactly what I wanted. Programs run with their media.role set to "browser" will make anything run with the media.role of "music" mute themselves. Implementing it into my environment was straight forward:
- load the module using: pacmd load-module module-role-ducking trigger_roles="browser" volume=0
- load the module on boot using: echo "load-module module-role-ducking trigger_roles="browser" volume=0" >>/etc/pulse/default.pa"
- add "PULSE_PROP='media.role=browser'" to any applications environment that I want to mute my music
- run cmus with the environment "PULSE_PROP='media.role=music'"
- patch urlopener to also run programs with "PULSE_PROP='media.role=browser'", see the commit here
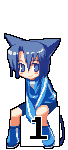
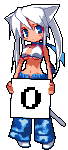
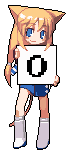
MariaDB hassle
3/11/2019
For a TAFE project I am doing some stuff in PHP (simple store, see it live on https://manga.gnupluslinux.com and find the source code here) and it requires some databases.
I designed the database in WorkBench and attempted to forward engineer it to my local instance of MariaDB which lead to an error message.
Long story short, MariDB does not support the 'VISIBLE' key word, yet it claims to be a drop in replacement for MySQL. It mostly is, but that's annoying.
You can either remove the keyword 'VISIBLE' from the generated script or set your target MySQL version to 5.7 in WorkBench which does not support the keyword.
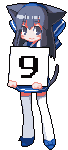
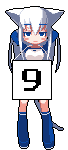
simple csv parse
30/10/2019
I store purchases I make in a spreadsheet. The spreadsheet is becoming a pain to use so I am creating a program to store them in a database and prettyprint purchases.
The first piece needed was to parse the csv output of the spreadsheet, I did this with a simple C program:
#define _GNU_SOURCE #include <stdio.h> #include <stdlib.h> #include <string.h> struct purchase { char *product; char *website; char *date; char *cost; char *notes; }; void parsepurchase(struct purchase *p, char *line) { char *modline; char *bup; size_t linesize; size_t len; linesize = strlen(line); modline = malloc(linesize + 1); bup = modline; strcpy(modline, line); modline = strtok(modline, ",\""); p->product = malloc(strlen(modline) + 1); strcpy(p->product, modline); modline = strtok(NULL, ",\""); p->website = malloc(strlen(modline) + 1); strcpy(p->website, modline); modline = strtok(NULL, ",\""); p->date = malloc(strlen(modline) + 1); strcpy(p->date, modline); modline = strtok(NULL, ",\""); p->cost = malloc(strlen(modline) + 1); strcpy(p->cost, modline); modline = strtok(NULL, ",\""); p->notes = malloc(strlen(modline) + 1); strcpy(p->notes, modline); /* remove unwanted newline when note exists */ len = strlen(p->notes) - 1; if (p->notes[len] == '\n') p->notes[len] = '\0'; printf("%s, %s, %s, %s, %s\n", p->product, p->website, p->date, p->cost, p->notes); free(bup); } void freepurchases(struct purchase *purchases, size_t count) { size_t i = 0; while (i != count) { free(purchases[i].product); free(purchases[i].website); free(purchases[i].date); free(purchases[i].cost); free(purchases[i].notes); i++; } } int main(int argc, char **argv) { FILE *file; struct purchase purchases[512]; /* max 512 entries for now */ char *line; size_t len; ssize_t read; int i; if (argc != 2) { printf("use: %s csvfile\n", argv[0]); } file = fopen(argv[1], "r"); if (!file) { fprintf(stderr, "unable to open csv file\n"); exit(EXIT_FAILURE); } line = NULL; len = 0; i = 0; while ((read = getline(&line, &len, file)) != -1) { parsepurchase(&purchases[i], line); i++; } freepurchases(purchases, i); fclose(file); free(line); return EXIT_SUCCESS; }
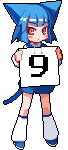
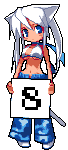
more updates
12/9/2019
UPS battery failing
My CyberPower BR650ELCD UPS battery finally had its battery die after many years, no idea how many, 4-5? I purchased a new battery for it.I also purchased a second UPS, the APC BX700U. It is generally the same specs, 700va 390W, though this one is not a user replaceable battery.
I've now put the CyberPower UPS upstairs for my camera server + router/modem and kept the APC in my room.
4-Bit Adder
A month or so ago I started building a 4-Bit Adder circuit on a breadboard using logic gates, I've only implemented 2 bits so far, education and work became busy. This was my first go at using solid-core wire, so it is pretty ugly.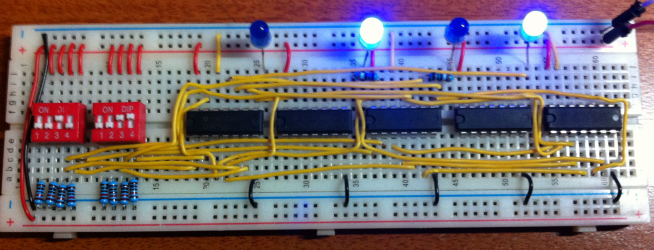
Manga
I also have my first shelf filled! These are the series I hold dearest to my SOL loving heart.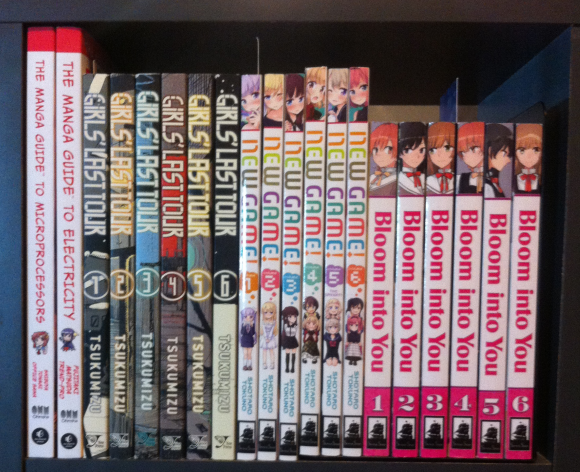
I don't know if I will continue getting manga or go towards artwork for a while, my walls are bare. I'd like to get some figurines, but quality ain't cheap. I've found many wall scrolls I'd like to get, I should commit to it.
old project
An old project of mine animegrep (which I named 'everytime-desu' originally) has been picked up by someone else and improved upon a little. You can find their fork here.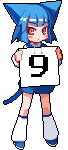
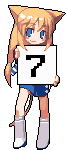
some things
14/7/2019
A while ago I started writing a project that I intended to implement into sic that would warn me when I attempt to repost a link into an IRC channel. My interest in the project faded, so I likely will never complete it.
The source code can be found in my git repo.
A month or two ago I pulled the plug on self-hosting my email and now use another provider. It isn't ideal, but whatever.
I started a manga collection and have very quickly amassed 19 volumes of series I loved. I also picked up a few volumes from the Manga guide to series. I'm currently reading the volumes on electricity and microprocessors. Very fun and educational.
I also a while ago acquired a couple stm32 dev boards that I intended to learn and use, problem being shipping took a long time and my interest bled, I need to find motivation for that again.
I am also quickly nearing 100 posts here, currently this is 97. Now that means nothing as half of it is dribble, but my sitegenerator by memory will break when it has to handle the 100th post. I hope my memory is wrong, I really don't want to look at that disgusting code again.
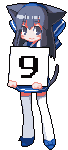
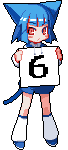
xwake
17/4/2019
I created a simple program in C that prevents the X11 screensaver from starting between two times. In hindsight cronjobs would have been easier.
The source code can be found in my git repo.
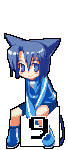
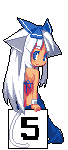
Product Inventory remake
11/4/2019
At the end of 2018 I was asked to create an inventory system for someone that wanted to document what they owned. They couldn't find a system that had the features they wanted. So I did create it, it works fine and is still active and functional today. However, the project is written in PHP and is a mess. Since then I've learnt a lot more about realtional databases and how to properly design them, so I recreated the entire system in C++ and Qt.
Currently the filtering options aren't implemented, but the table generation, category loading and database management settings work.
The entire interface in the filter tab
The database tab
The source code can be found in my git repo.
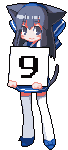
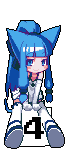
X bell listener
26/3/2019
I wanted to be able to run a script when the X bell is rung, so I made a program that listens for the X bell notify event and runs a user defined program when it is caught.
The source code can be found in my git repo.